Below is the code that can be used, and there are a two changes to note that have come with the iOS 8 SDK. The first is UIImagePickerControllerSourceType where before you would access it by calling UIImagePickerControllerSourceTypeCamera now you call UIImagePickerControllerSourceType.Camera . The second has more to do with strings different types, CFString vs NSString so to deal with this little issue the following code exists:
let compResult:CFComparisonResult = CFStringCompare(mediaType as NSString!, kUTTypeImage, CFStringCompareFlags.CompareCaseInsensitive)
if ( compResult == CFComparisonResult.CompareEqualTo ) {
Other than that there are the obvious differences between Objective-C and Swift. I hope this helps.
Example Code:
import UIKit
import CoreData
import MobileCoreServices
class CameraVC: UIViewController,UINavigationControllerDelegate,UIImagePickerControllerDelegate {
@IBOutlet weak var cameraView: UIImageView!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
override func viewWillAppear(animated: Bool) {
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
// MARK: - Action Methods
@IBAction func takePhoto(sender: AnyObject) {
if (UIImagePickerController.isSourceTypeAvailable(UIImagePickerControllerSourceType.Camera)){
var picker = UIImagePickerController()
picker.delegate = self
picker.sourceType = UIImagePickerControllerSourceType.Camera
var mediaTypes: Array<AnyObject> = [kUTTypeImage]
picker.mediaTypes = mediaTypes
picker.allowsEditing = true
self.presentViewController(picker, animated: true, completion: nil)
}
else{
NSLog("No Camera.")
}
}
// MARK: - Delegate Methods
func imagePickerController(picker: UIImagePickerController, didFinishPickingMediaWithInfo info: NSDictionary!) {
NSLog("Did Finish Picking")
let mediaType = info[UIImagePickerControllerMediaType] as String
var originalImage:UIImage?, editedImage:UIImage?, imageToSave:UIImage?
// Handle a still image capture
let compResult:CFComparisonResult = CFStringCompare(mediaType as NSString!, kUTTypeImage, CFStringCompareFlags.CompareCaseInsensitive)
if ( compResult == CFComparisonResult.CompareEqualTo ) {
editedImage = info[UIImagePickerControllerEditedImage] as UIImage?
originalImage = info[UIImagePickerControllerOriginalImage] as UIImage?
if ( editedImage == nil ) {
imageToSave = editedImage
} else {
imageToSave = originalImage
}
NSLog("Write To Saved Photos")
cameraView.image = imageToSave
cameraView.reloadInputViews()
// Save the new image (original or edited) to the Camera Roll
UIImageWriteToSavedPhotosAlbum (imageToSave, nil, nil , nil)
}
picker.dismissViewControllerAnimated(true, completion: nil)
}
func imagePickerControllerDidCancel(picker: UIImagePickerController) {
picker.dismissViewControllerAnimated(true, completion: nil)
}
}
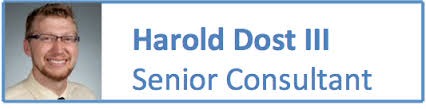
No comments:
Post a Comment